C++
문자열
yoosorang
2025. 2. 9. 12:41
🔹 문자열 연결
#include <iostream>
#include <string>
using namespace std;
int main() {
string str1 = "Hello, ";
string str2 = "World!";
//1. + 연산자
string result = str1 + str2;
cout << result << endl;
//2. append() 함수 사용
str1.append(str2);
cout << str1 << endl;
return 0;
}
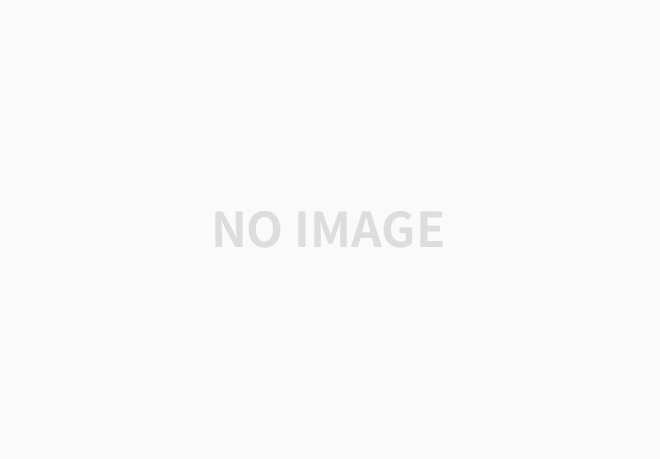
🔹 문자열 대체
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "Hello World";
string newStr = "C++ Programming";
// 1. 인덱스 기준 대체
str.replace(6, 5, "C++");
// 출력: Hello C++
// 2. 반복자 사용하여 대체
str.replace(str.begin() + 6, str.begin() + 11, newStr.begin(), newStr.end());
// 출력: Hello C++ Programming
//3. 문자열 일부를 다른 문자열의 일부로 대체
str1.replace(6, 5, newStr, 0, 3); // newStr의 0번째부터 3글자만 사용
// 출력: Hello C++
cout << str << endl; // 출력: Hello C++
return 0;
}
🔹 이스케이프 시퀀스
백슬래시(\\)로 시작하는 문자 조합으로 문자열에 특수 문자나 특정 동작(줄바꿈, 탭 등)을 포함 가능
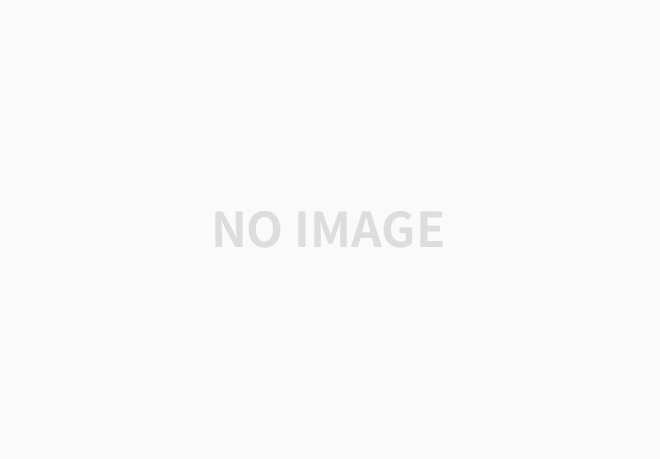
🔹 Raw 문자열 리터럴
이스케이프 시퀀스를 사용하지 않고 괄호 안에 들어간 문자열을 그대로 출력
#include <iostream>
using namespace std;
int main(void) {
cout << R"(!@#$%^&*(\\'"<>?:;)";
}